Modules
https://github.com/golang/go/wiki/Modules
Table of Contents
- Quick Start Example
go.mod
- Semantic Import Versioning
- Define a Module
- Prepare for a Release
- Opening keynote: Go with Versions - GopherConSG 2018
Quick Start Example tutorial
$ mkdir -p ~/temp/hello
$ cd ~/temp/hello
$ go mod init temp/hello
go: creating new go.mod: module github.com/you/hello
module temp/hello
require rsc.io/quote v1.5.2
go.mod
reference
- Modules must be semantically versioned in the form
v(major).(minor).(patch)
, such asv0.1.0
,v1.2.3
, orv3.0.1
- If using Git, tag released commits with their versions.
- There are four directives:
module
,require
,exclude
,replace
. exclude
andreplace
directives only operate on the current (“main”) module.
All of the packages in a module share a common prefix – the module path. The
go.mod
file defines the module path via the module directive. For example, if you are defining a module for two packagesexample.com/my/project/foo
andexample.com/my/project/bar
, the first line in yourgo.mod
file typically would be moduleexample.com/my/project
, and the corresponding on-disk structure could be:
project/
├── go.mod
├── bar
│ └── bar.go
└── foo
└── foo.go
Semantic Import Versioning discussion
- Follow semantic versioning (with tags such as
v1.2.3
). - If the module is version v2 or higher, the major version of the module must be included in both the module path in the go.mod file (e.g., module
example.com/my/mod/v2
) and the package import path (e.g.,import "example.com/my/mod/v2/foo"
). - If the module is version
v0
orv1
, do not include the major version in either the module path or the import path.
Define a Module howto
Most projects will follow the simplest approach of using a single module per repository, which typically would mean creating one go.mod file located in the root directory of a repository.
Prepare for a Release howto
- Run
go mod tidy
to possibly prune any extraneous requirements - Run
go test all
to test your module - Ensure your
go.sum
file is committed along with yourgo.mod
file.go.sum
contains the expected cryptographic checksums of the content of specific module versions.- Note that
go.sum
is not a lock file as used in some alternative dependency management systems.
Opening keynote: Go with Versions - GopherConSG 2018 tutoral
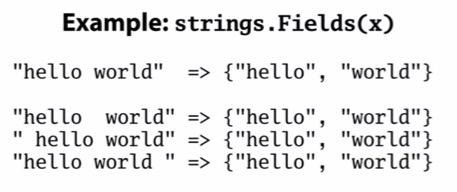
strings.Split()
, introduce a new name for a new meaning.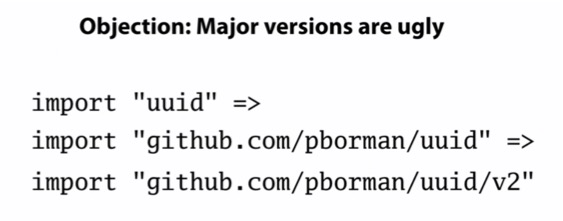
name
to github.com/owner/name
, people will get used to it.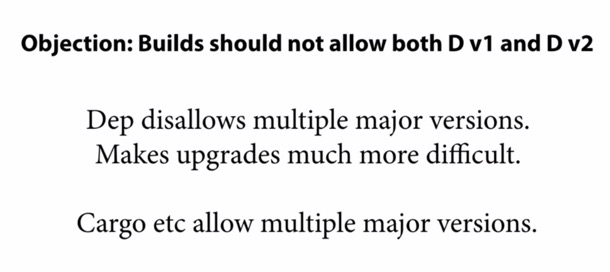
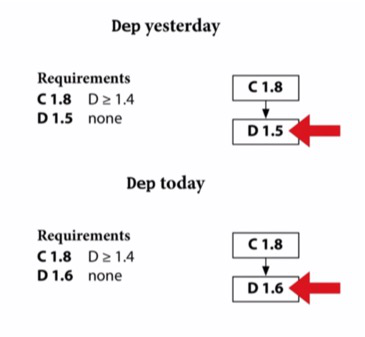
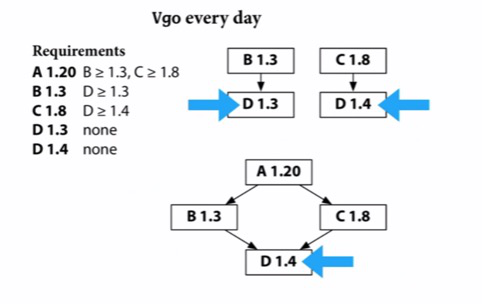
vgo
selects the minimum available version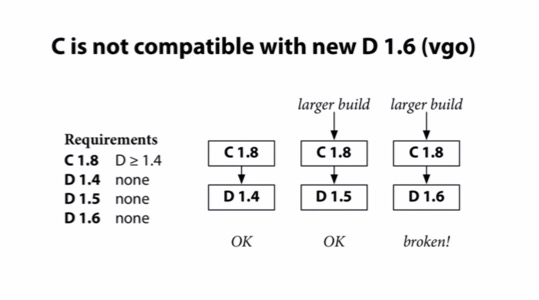
D 1.6
released. For some reason, C 1.8
and D 1.6
do not work correctly. Regardless of which package caused the problem, users won't be affected by this Because C 1.8
has not yet released.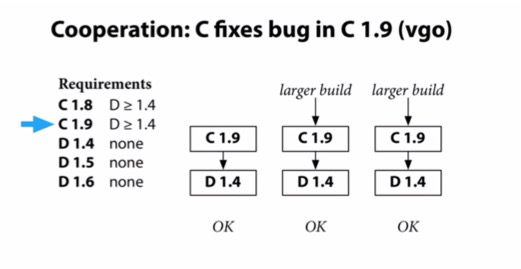
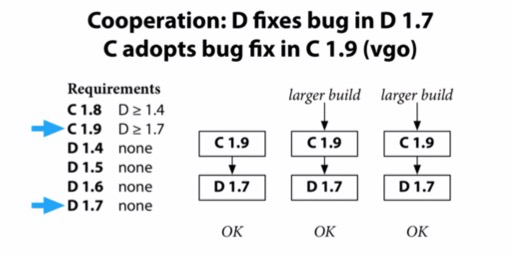
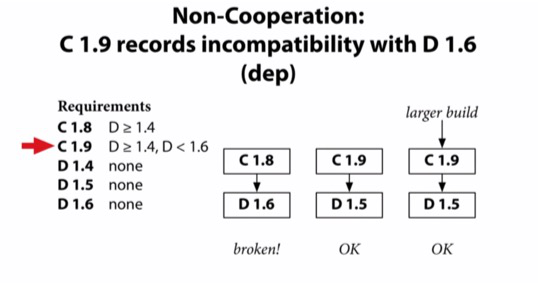
dep
uses, which picks the latest available version, the problematic C 1.8
and D. 1.6
combo will be exposed to users. So, to deal with this emergency situation, the author of C
had to release another C 1.9
with the upper bound verson of D
.- The reasoning of the policy
- Packages should support backward minor-version compatibility.
- When there is a conflict, pick the minimum available version.
- Unless there is a version inconsistency, like cyclic dependencies or a situation in which the program cannot determine the minimum common version, even when some dependencies are updated, the compatibility and repeatability are maintained.